Development environment
- Django==4.1.2 Python 3.10.6
- Using the Django authentication system
- No design addes
Create a Superuser
Very first user to create is Superuser (you).
Open the terminal and create one.
python3 manage.py createsuperuser
Username: admin
Email address: admin@example.com
Password: **********
Password (again): *********
Superuser created successfully.
Run server (python3 manage.py runserver) and login to admin panel to make sure you can login
URL: 127.0.0.1.8000/admin
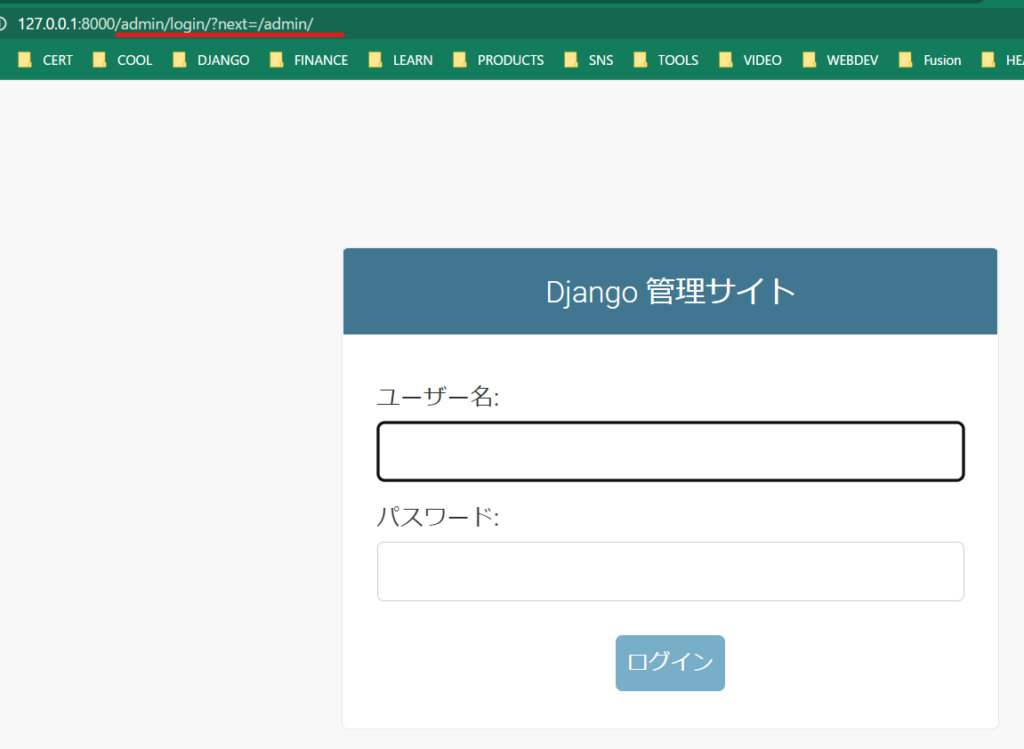
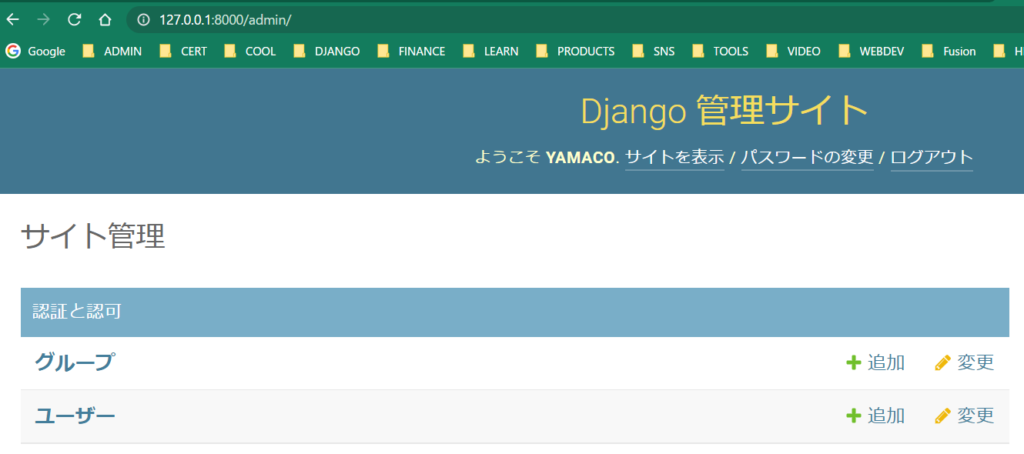
Click User (ユーザー)to show users
You’ll find your name as a superuser who has all permissions
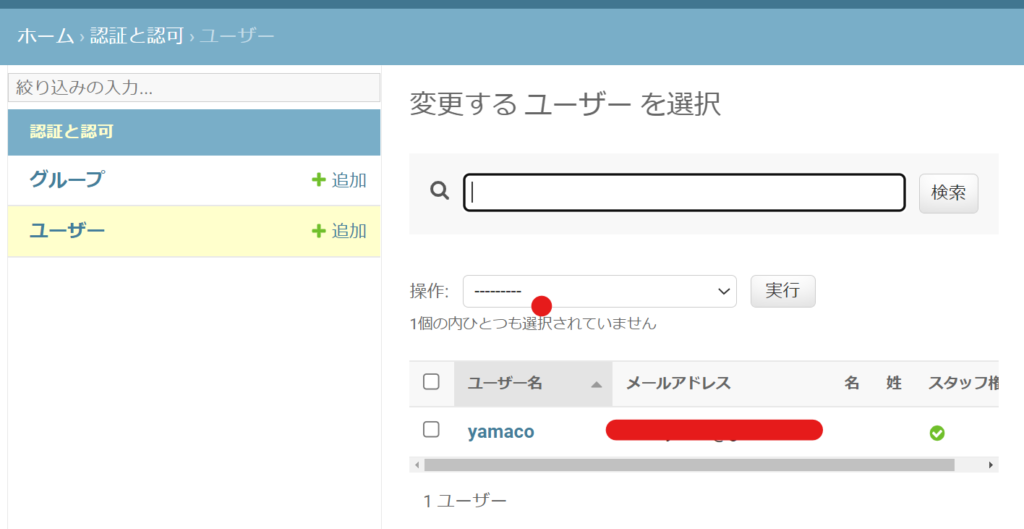
Folder structure
Create a template folder inside the user app (not inside the project)
Create base.html directly under the template folder
Create a registration folder and a user folder directly under the template folder
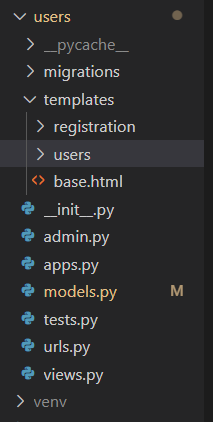
Create login.html in registration folder
create dashboard.html in users folder
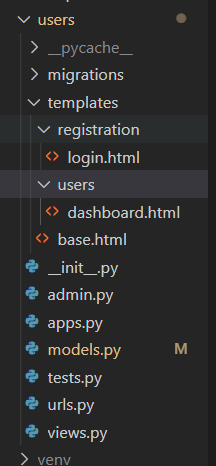
User Dashboard
On the first line add {% extends ‘base.html’ %} to show contents of dashboard.html on base.html.
By default, user.username = “”, so let’s put ‘Teacher’ when not logged in
You can change contents to show depending on login status.
<!-- Make sure add this code to send content to base.html -->
{% extends 'base.html' %}
<!-- Content on this template will be shown on base.html -->
{% block content %}
<!-- User without login will see this message -->
Hello, {{ user.username|default:'Teacher' }}!
<!-- Display different messages according to login status -->
<div>
{% if user.is_authenticated %}
<a href="{% url 'logout'%}">Logout</a>
{% else %}
<a href="{% url 'login' %}">Login</a>
{% endif %}
</div>
{% endblock %}
What to write on base.html
Add simple style to base.html
{% block content %}{% endblock %} part will be used for populating contents from other templates.
<h1>This is a Student Report</h1>
<!-- Add simple style to login page -->
<style>
label, input{
display: block;
}
span.helptext{
display: none;
}
</style>
<!-- This is where contents will be fed from other templates -->
{% block content %}
{% endblock %}
Create users/templates/registration/login.html
Very simple login page.
This time again, not to forget to add {% extends ‘base.html’ %} on the first line.
Also, it’s very important to add {% csrf_token %} to the form to make it safe.
Django has a {% csrf_token %} tag that is implemented to avoid Cross-Site Request Forgery (CSRF) attacks.
What is a CSRF token in Django?
{% extends 'base.html' %}
{% block content %}
<form method="post">
# csrf_token is Cross Site Request Forgery protection
{% csrf_token %}
# Display the contents of the form by enclosing them in p tags
{{ form.as_p }}
<input type="submit" value="Login">
</form>
# Link takes users back to dashboard
<a href="{% url 'dashboard' %}">Back to dashboard</a>
{% endblock %}
FYI, in django, besides form.as_p, there are other convenient ways to write forms in html files.
ex1: from.as_table(). This encloses the contents of the form in tags.
ex2: It is form.as_ul. This encloses the contents of the form in tags.
views.py to specify what to show
Now, specify what you want to show by adding code to users/views.py
On the 1st line, you import render method from django.shortcuts for rendering templates.
def the view for dashboard.html
# Renders the specified template and returns an HttpResponse
from django.shortcuts import render
# Render and return the contents of dashboard.html
def dashboard(request):
return render(request, "users/dashboard.html")
Now that we specified the view, we can call it on urls.py
urls.py for mapping URLs
We have to tell both project/urls.py and app/urls.py for mapping URL.
(In this case, students/urls.py and users/urls.py)
We need to tell Django the urls by adding the view to users/urls.py
Django maps the view URL in each application’s urls.py and include each application’s urls.py in the project’s urls.py, so first add the include method.
Then, describe which views to include in the URL.
from django.urls import path, include
from users.views import dashboard
urlpatterns = [
# Add django.contrib.auth
path("accounts/", include("django.contrib.auth.urls")),
# Add path to dashboard
path("dashboard/", dashboard, name="dashboard"),
]
Write the above path in urls.py of the project
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
# Include users.urls to the path
path('', include('users.urls')),
path('admin/', admin.site.urls),
]
Redirect URL to settings.py
Let’s add redirect page when logged in and logged out to students/settings.py
(There are several ways to redirect other than the above)
You can add them at the bottom of the file
# Redirect to "dashboard.html" when logged in
LOGIN_REDIRECT_URL = "dashboard"
# Redirect to "dashboard.html" when logged out
LOGOUT_REDIRECT_URL = "dashboard"
Outcome Pages
When logged out “Login” link will show
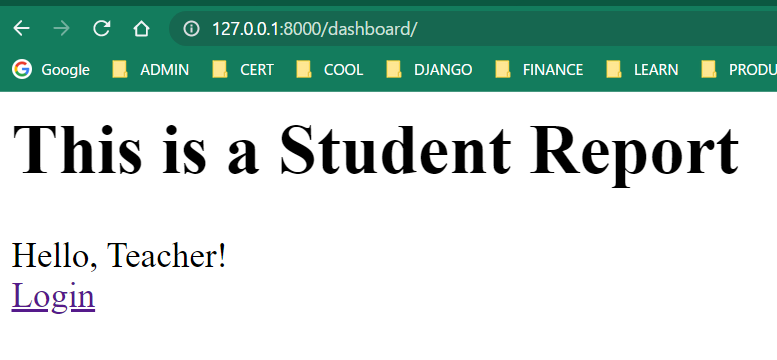
After checking your name on admin panel, go back to dashboard and click “Login”
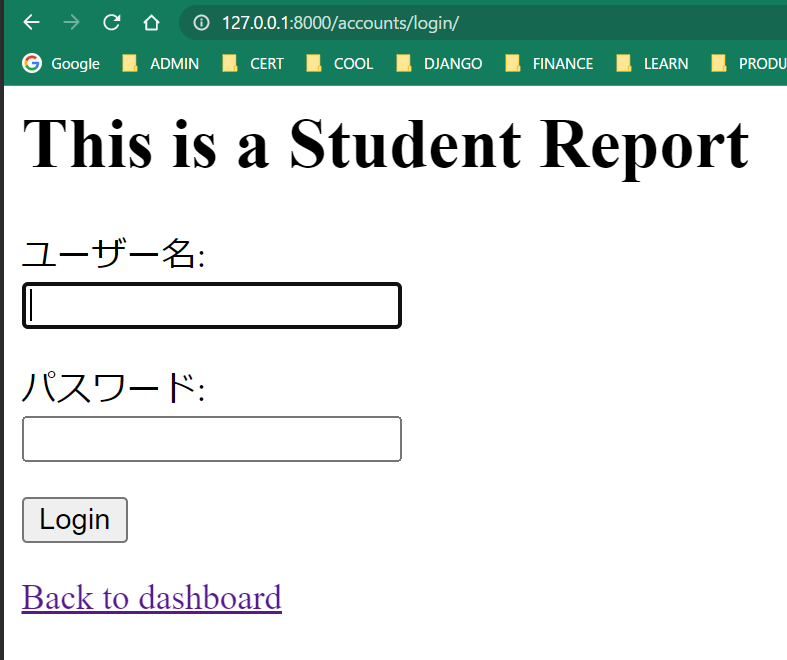
When logged in, “Logout” message will show
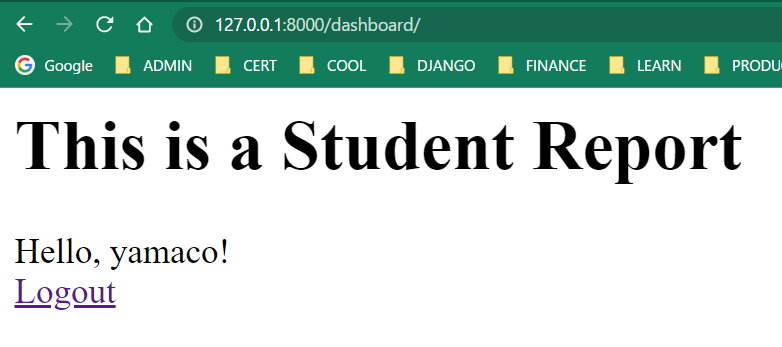