A central feature of Django is the Admin Panel.
In this article, I will describe how to customize the Admin Panel and make it more convenient.
What is a Django Admin Panel in the first place?
In a nutshell, the Admin Panel is a Django built-in application that provides CRUD (Create, Read, Update, Delete) screens for records corresponding to models.
django.contrib.admin, which is in the INSTALLED_APPS in settings.py, is the Django admin site. This is enabled by default.
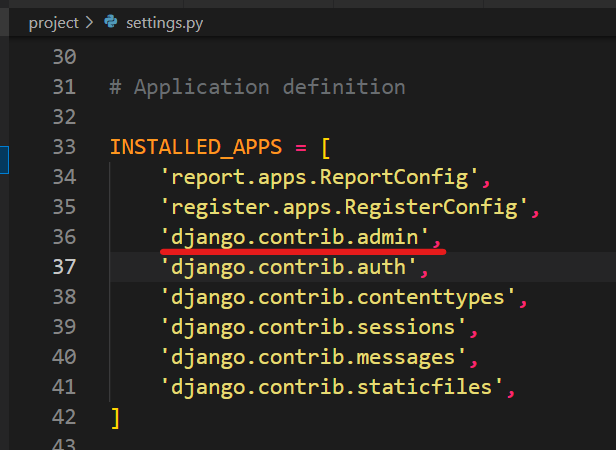
Login Condition
As for login, the Django Admin Panel uses the user model by default. However, it can also be a custom user model. In that user model, the login condition is “both the is_staff and is_active attributes are True”. You can customize this condition. I will introduce it later.
Change the name of each record displayed on the list
Below is the record list.
Here, a model called Course was created as an example.
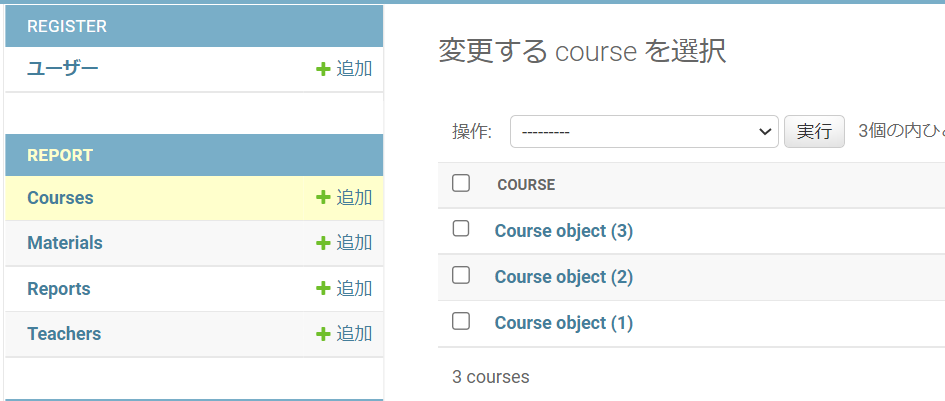
On the record list screen above, Course object (1), Course object (2), etc. are displayed, and this time I will explain how to change these names.
Simply add __str__ to the following models
## Before Change
class Course(models.Model):
course_name = models.CharField("Course", max_length=30, blank=True)
Addig
def __str__(self):
return self.course_name
will do the trick.
## After Change
class Course(models.Model):
course_name = models.CharField("Course", max_length=30, blank=True)
def __str__(self):
return self.course_name
Now, you can see the names of each object.
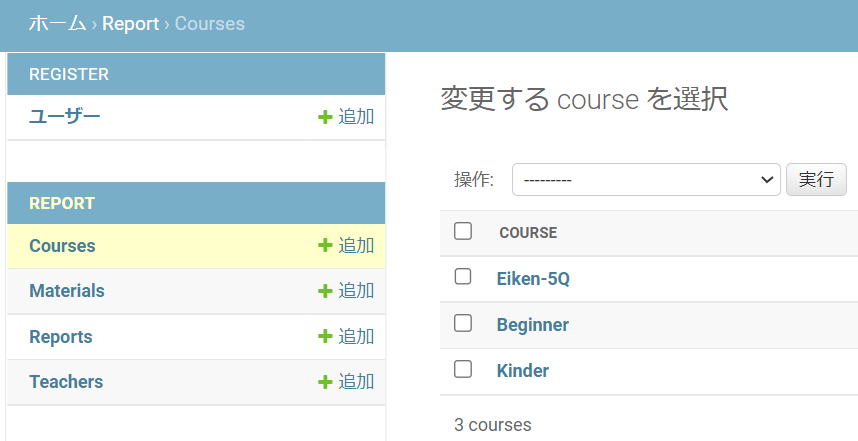
In case you want to display more than 1 item, use f-string like:
return f”{self.student_name}/ course: {self.course}/ email: {self.user}/ material: {self.material}”
## In case you want to display more than 1 item
class Report(models.Model):
student_name = models.CharField("生徒名", max_length=30)
course = models.ForeignKey(Course, verbose_name='コース', on_delete=models.PROTECT, default=1,)
user = models.OneToOneField(settings.AUTH_USER_MODEL, on_delete=models.PROTECT)
material = models.ManyToManyField("Material",)
def __str__(self):
return f"{self.student_name}/ course: {self.course}/ email: {self.user}/ material: {self.material}"
Change display items and order
Next, let’s change the display items and the order of the items (columns) on the list.
This can be done by setting a class that inherits ModelAdmin to each model in admin.py as follows.
With fields = [‘course’, ‘category’, ‘title’, ‘unit’], changing the order in parentheses changes the input order
# Create a class that inherits from django.contrib.admin.ModelAdmin
class MaterialAdmin(admin.ModelAdmin):
# set the fields variable
fields = ['course', 'category', 'title', 'unit']
# Set to the second argument of admin.site.register
admin.site.register(Material, MaterialAdmin)
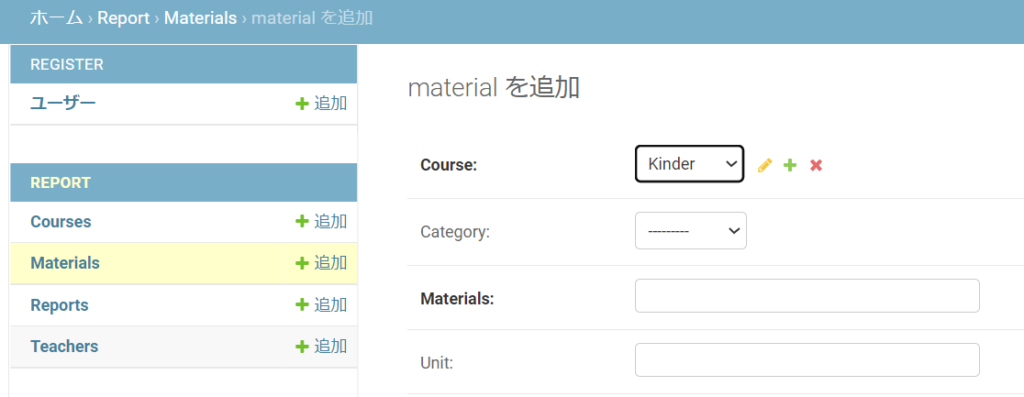
Change the search target on the record list
Add search_fields with target words: [‘course’, ‘category’]
# Create a class that inherits from django.contrib.admin.ModelAdmin
class MaterialAdmin(admin.ModelAdmin):
# set the fields variable
fields = ['course', 'category', 'title', 'unit']
# Add search_fields and target words
search_fields = ['course', 'category']
# Set to the second argument of admin.site.register
admin.site.register(Material, MaterialAdmin)
When searching, middle match search is performed from [‘course’, ‘category’]
Search box added
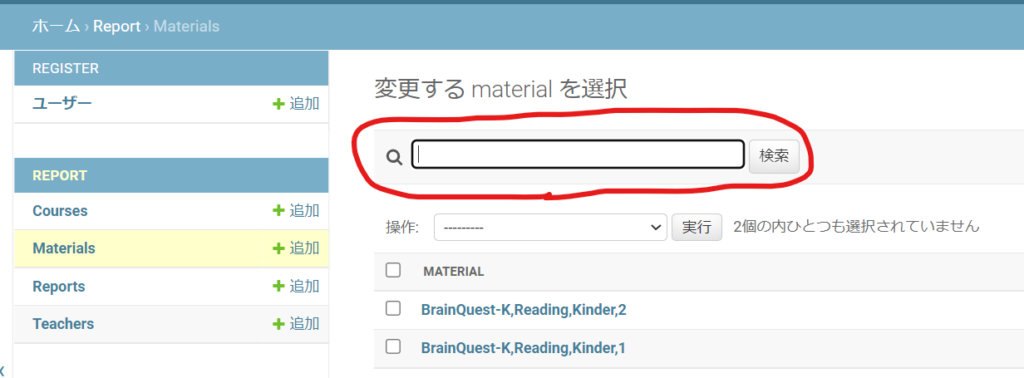
Add a filter on the record list
Add list_fileter to admin.py for filtering records with the same element.
# Create a class that inherits from django.contrib.admin.ModelAdmin
class MaterialAdmin(admin.ModelAdmin):
# set the fields variable
fields = ['course', 'category', 'title', 'unit']
# Add search_fields and target words
search_fields = ['course', 'category']
# Add filter bar on the list
list_filter = ['course', 'category', 'title']
# Set to the second argument of admin.site.register
admin.site.register(Material, MaterialAdmin)
Filter has been added.
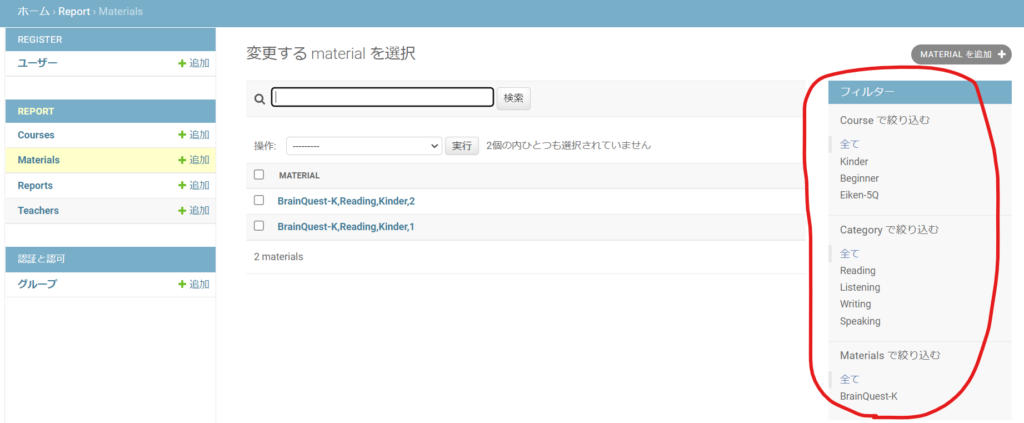
Make it possible to edit the value on the list
To edit the value on the list, set list_editable in admin.py.
Also, columns set to list_editable must be set to list_display.
Since the first column of list_display will be the link of the detail, it cannot be set to list_editable (however, the second and subsequent columns can be set in list_display_links)
Now, Material is editable on the list
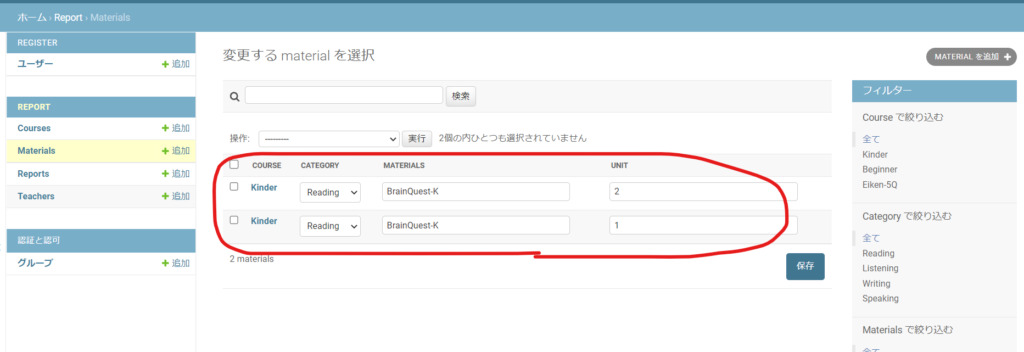
When setting ‘COURSE’ as list_editable, then list_display_links needs to be specified:
# list_editable = [‘course’]
# list_display_links = [‘category’]
Django Admin – Redesign and Customization
Basic Routing is explained here: