Display the Typing Game board
The first step is to learn the basics of pygame.
import pygame as pg
import sys
pg.init()
BOARD = pg.display.set_mode((400, 300))
pg.display.set_caption('Learn Japanese Game')
while True:
for event in pg.event.get():
if event.type == pg.QUIT:
pg.quit()
sys.exit()
pg.display.update()
When the above code is executed, the board will appear as shown below and to the right.
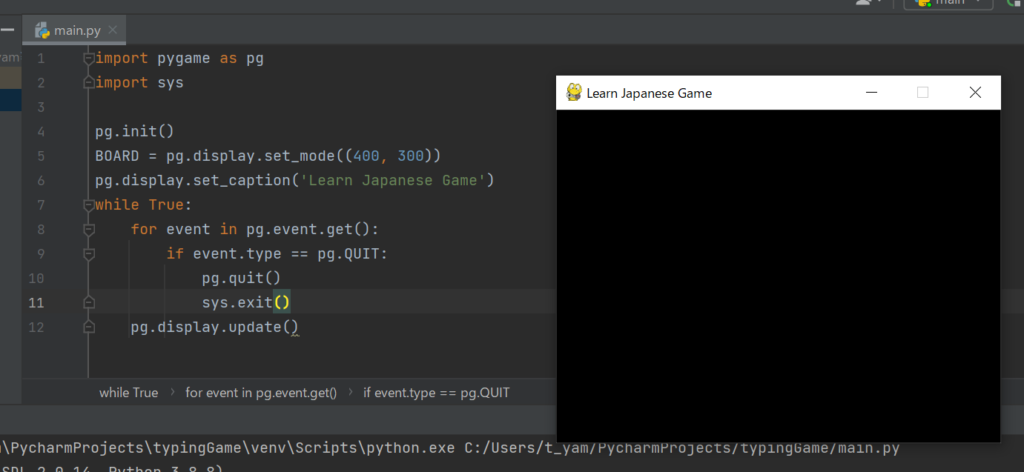
- Set the display size in BOARD.
- Display board title with set_caption.
- In a while loop, the following three steps are repeated.
1. Handles events.
2. Updates the game state.
3. Draws the game state to the screen.
- pg.quit() stops the pygame library and must be written before sys.exit().
- pygame.display.set_mode() returns the board to the initial screen.
Pixel coordinates and drawing
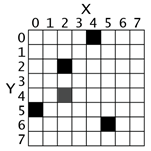
Get color code with RGB Color Picker
Color the board and draw a rectangle.
Specify location and size with pg.draw.rect(board, color, top-left x, top-left y, width, height).
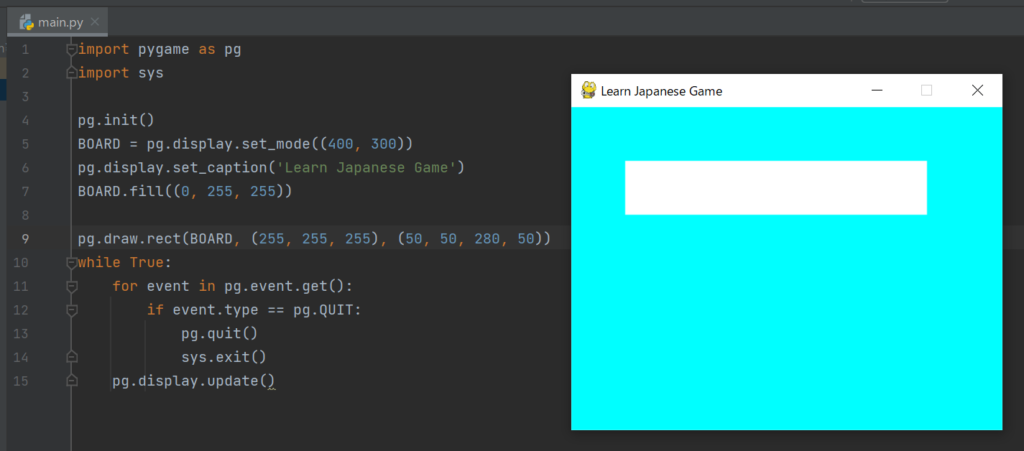
Typing Game Setup Start
Summarize the features required for the game
- game screen
- Game start, game reset
- Area to display the subject and the text you entered.
- Typing speed measurement
- Percentage of typing correct
- Result display (elapsed time, percentage of correct answers, words per minute)
New words and references learned this time
pygame = A game library for creating 2D games in Python.
reference:【Pygame入門】ゲームプログラミング【Python】
reference2:pygame公式サイト
reference3:Making Games with Python & Pygame
dpi = Mouse Sensitivity。
reference:マウスのDPIを調整して使い勝手を向上!
enumerate = Using the enumerate function, an element’s index and element can be retrieved at the same time
pygame.mouse = Various settings can be configured for mouse operation.
pygame.mouse.get_pos
()get the mouse cursor positionget_pos() -> (x, y)Returns the
pygame documentationx
andy
position of the mouse cursor.
clock.tick() = This instruction is executed every frame. The number of milliseconds since the last execution of this instruction is calculated and returned as the return value.